Last updated on August 5th, 2023
Introduction
GoFiber is a fast, lightweight, and flexible web framework for Go (Golang), while GORM is a powerful Object-Relational Mapping library that simplifies database interactions. In this article, we will explore how to perform CRUD (Create, Read, Update, Delete) operations using GoFiber and GORM with a MySQL database. Additionally, we will emphasize the importance of code reusability to make the application more maintainable and scalable.
Prerequisites
Before we dive into the code, ensure you have the following installed on your machine:
- Go programming language: Official Website
- MySQL database: Official Website
Setup GoFiber and GORM Project Steps
- Create a GoFiber and GORM project
First, let’s set up a new GoFiber project. Open a terminal and run the following command:
go mod init <your_project_name>
go get -u github.com/gofiber/fiber/v2
go get -u gorm.io/gorm
go get -u gorm.io/driver/mysql
- Import required packages
In your project’s main.go file, import the necessary package, routes will define later:
package main
import (
"github.com/gofiber/fiber/v2"
)
- Create database connection
Next, we’ll create a function to connect to the MySQL database. Replace the connection details with your MySQL credentials:
package main
import (
"fmt"
"gorm.io/driver/mysql"
"gorm.io/gorm"
"log"
)
var db *gorm.DB
func connectDB() {
dsn := "user:password@tcp(127.0.0.1:3306)/database_name?charset=utf8mb4&parseTime=True&loc=Local"
var err error
db, err = gorm.Open(mysql.Open(dsn), &gorm.Config{})
if err != nil {
log.Fatalf("failed to connect to database: %v", err)
}
fmt.Println("Database connected!")
}
- Define a reusable model structure
To maintain code reusability, let’s create a separate file called models.go
to define the model structure. For example, if we’re building a simple blog application, the structure could be:
package main
import "gorm.io/gorm"
type Post struct {
gorm.Model
Title string `json:"title"`
Content string `json:"content"`
}
- Implement CRUD operations
Now, let’s create a file named crud.go
where we’ll implement the CRUD operations:
package main
import (
"github.com/gofiber/fiber/v2"
)
func CreatePost(c *fiber.Ctx) error {
var post Post
if err := c.BodyParser(&post); err != nil {
return err
}
result := db.Create(&post)
if result.Error != nil {
return result.Error
}
return c.JSON(post)
}
func GetPosts(c *fiber.Ctx) error {
var posts []Post
db.Find(&posts)
return c.JSON(posts)
}
func UpdatePost(c *fiber.Ctx) error {
id := c.Params("id")
var post Post
db.First(&post, id)
if err := c.BodyParser(&post); err != nil {
return err
}
db.Save(&post)
return c.JSON(post)
}
func DeletePost(c *fiber.Ctx) error {
id := c.Params("id")
var post Post
db.Delete(&post, id)
return c.SendString("Post successfully deleted")
}
- Implement routing
Now, let’s define the routes in the main.go
file to call the CRUD functions:
package main
import (
"github.com/gofiber/fiber/v2"
)
func main() {
connectDB()
app := fiber.New()
// Routes
app.Post("/post", CreatePost)
app.Get("/posts", GetPosts)
app.Put("/post/:id", UpdatePost)
app.Delete("/post/:id", DeletePost)
// Start server
app.Listen(":3000")
}
- Final folder structure as below
gocrud/
├── main.go
├── db.go
├── models.go
└── crud.go
- Run the application
Finally, start the server by running:
go run .
when you will hit this command get similar output:
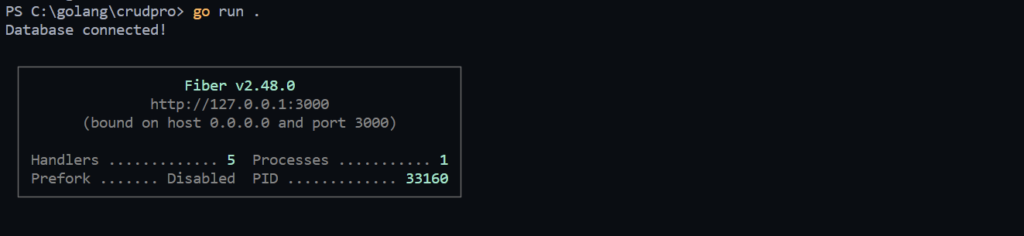
Table structure for application:
-- Table structure for table `posts`
--
CREATE TABLE `posts` (
`id` int(10) NOT NULL,
`title` varchar(255) NOT NULL,
`content` text NOT NULL,
`created_at` datetime NOT NULL DEFAULT current_timestamp(),
`updated_at` datetime NOT NULL DEFAULT current_timestamp(),
`deleted_at` datetime DEFAULT current_timestamp()
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_general_ci;
-- Indexes for table `posts`
--
ALTER TABLE `posts`
ADD PRIMARY KEY (`id`);
-- AUTO_INCREMENT for table `posts`
--
ALTER TABLE `posts`
MODIFY `id` int(10) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=3;
COMMIT;
Application Outputs and Screenshots
Now, let’s see the outputs of our application in action!
- Creating a New Post:
To create a new post, make a POST request to the “/post” endpoint with a JSON payload containing the “title” and “content” of the post. For example:
{
"title": "My new post",
"content": "This is blog content"
}

- Retrieving All Posts:
To retrieve all posts, make a GET request to the “/posts” endpoint. The server will respond with a JSON array containing all the posts.
Screenshot:

- Updating a Post:
To update a post, make a PUT request to the “/post/{id}” endpoint, where “{id}” is the ID of the post you want to update. Provide a JSON payload with the updated “title” and “content” of the post.
Screenshot:

- Deleting a Post:
To delete a post, make a DELETE request to the “/post/{id}” endpoint, where “{id}” is the ID of the post you want to delete.
Screenshot:

Conclusion
In this article, we explored how to perform CRUD operations using GoFiber and GORM with a MySQL database. By creating a reusable model structure and separating the database logic, we ensure code reusability, making our application more maintainable and scalable. Now you can build powerful web applications with GoFiber and GORM, taking advantage of their speed, flexibility, and ease of use. Happy coding!